A: Creating/Running a Command
Writing a Command Function
A command function in Harmony is simply a Dino function with a specific signature. The function name starts with the letters "cmd" (lowercase). It can be defined with any number of parameters. The purpose of a command function is to provide code that the user can execute on demand.
After we have saved our new rule script, we can move to the Editor tab to type in Dino code.
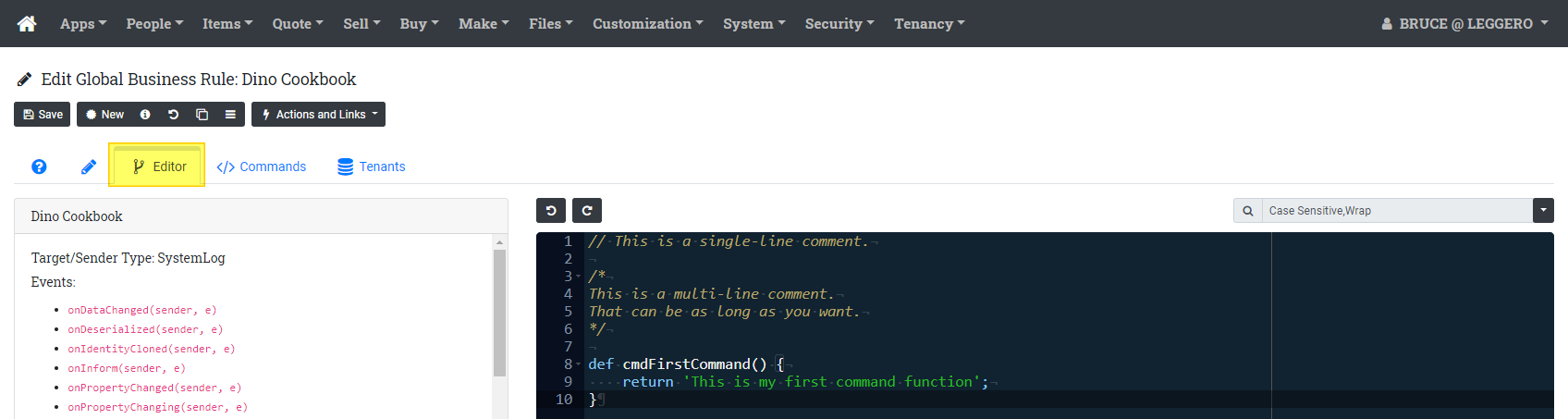
Here is our first command function:
/**
Command function that takes no arguments.
*/
def cmdFirstCommand() {
return 'This is my first command function';
}
So now we have our command function defined (don't forget to save the script record). But remember as we said above, the purpose of a command function is to provide dynamic code that the user of the system can execute on demand. So how do we allow the user to execute this code? They will need to be able to click on something to initiate the command, so how and where does it show up?
Creating a Command Record
Now that we have our command function (defined in the script), we need to add the command record, which is a separate data record. Switch to the Commands tab and press the Create New Command menu item (under the + menu) to add a new command record.

Set the Command Name (can be anything you want), select the Function Name (notice that the functions that start with "cmd" in the rule script will show up in a drop-down list here), and set the Context to List (will explain this later).
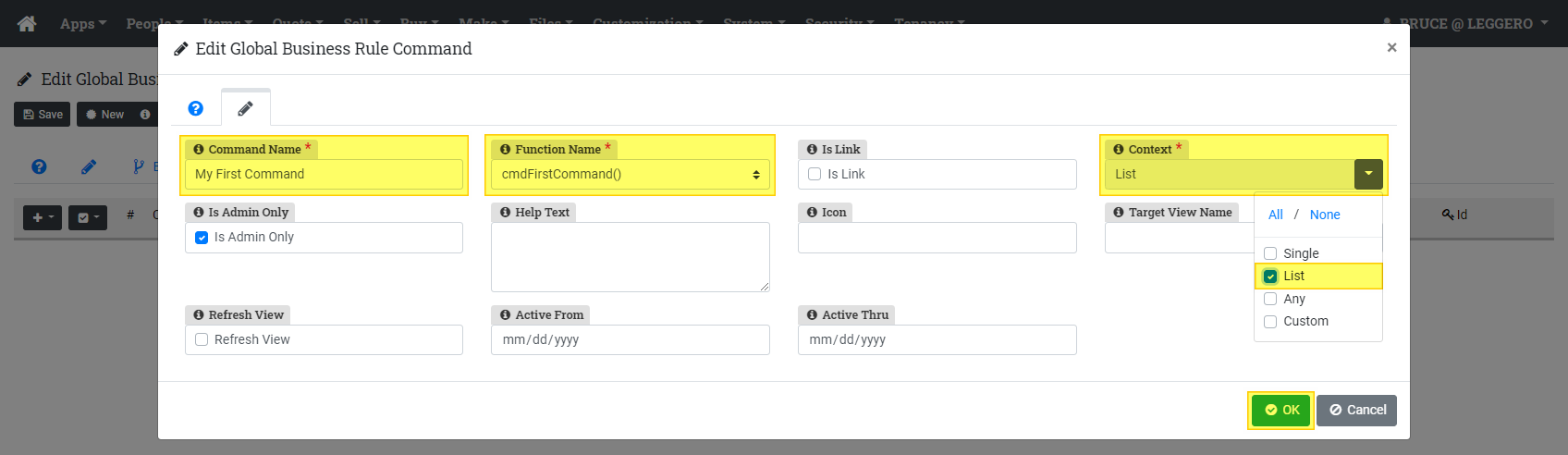
Press the OK button to add the command record to the list of commands available for our Dino Cookbook. Once the popup dialog disappears, press the Save button to save the rule script.
The list of commands available in the Dino Cookbook should look something like this:

Running a Command
Now, find the System Log menu entry. In our example system here, it is under the System menu.
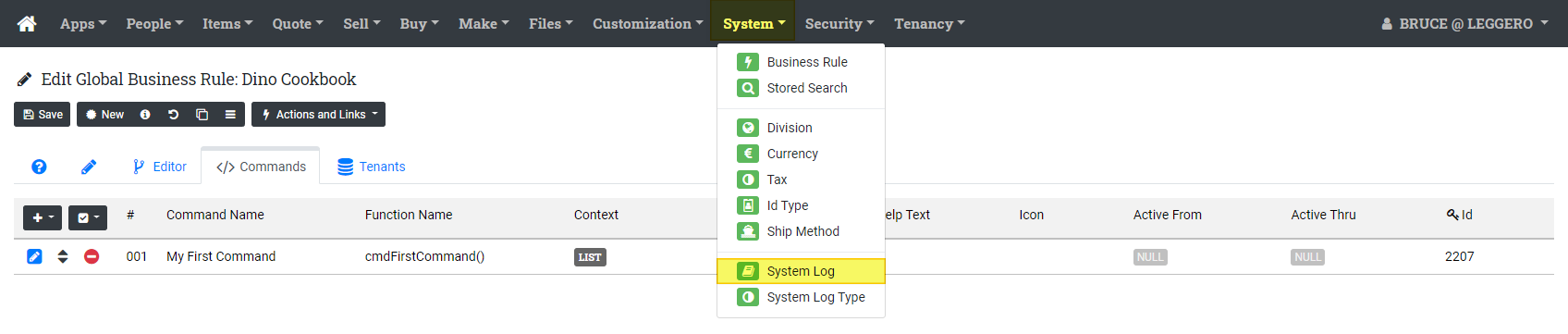
When you arrive at the System Log list page, click on the Actions and Links menu. It should show our new command!
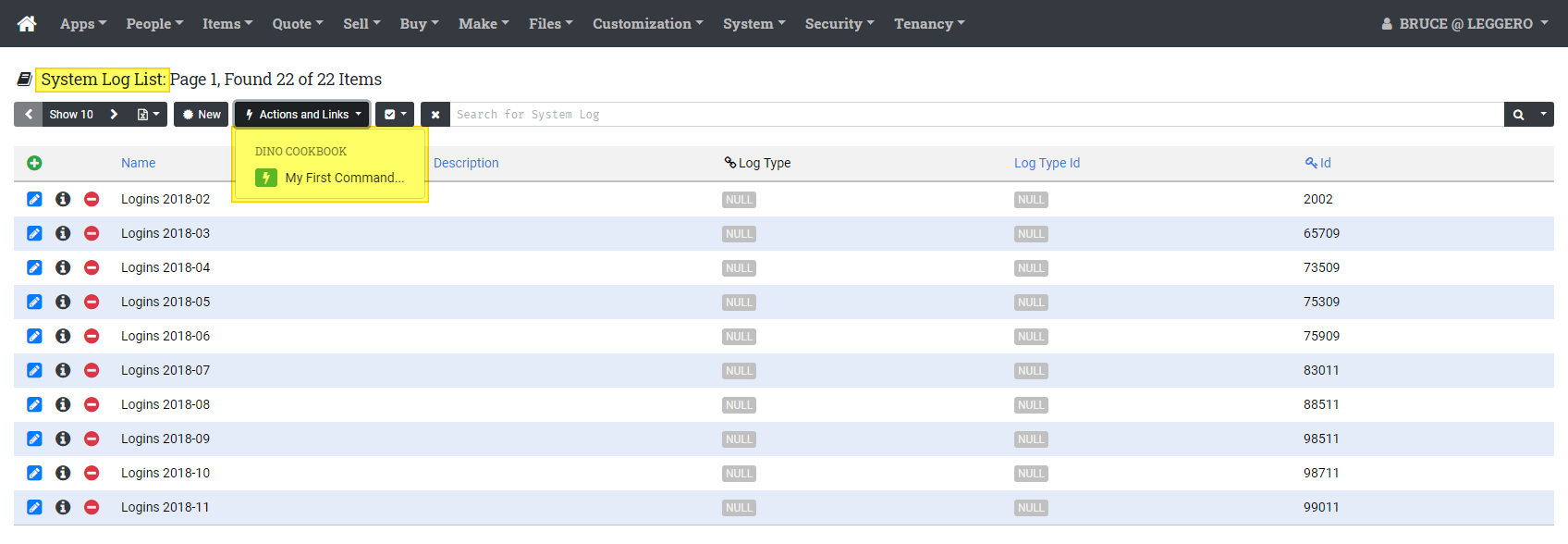
Let's recap how we got this to happen:
- We created a rule script record called Dino Cookbook. We set the Type Key (or Applies To) property of this record to the System Log type.
- We went to the Editor tab and entered a function starting with the letters "cmd".
- We went to the Commands tab and created a command record called "My First Command" that linked to the cmdFirstCommand function via a drop-down list.
- We set the Context of the command record to List.
- We then went to the System Log list page, where we see our command. The type of the records here we are looking at is System Log, and the context of the records we are looking at is the list context.
Click on the My First Command menu item to execute the cmdFirstCommand function.

Notice the message that says (No parameters required). This means that no input from the user is required to run this command. Click the Run button. The results of the command will show up in the body area of a popup dialog. For this command, we just returned a message as a simple string.

Default Return Value of a Command Function
If a return statement is not encountered in a Dino function def, it will always return the null value. In a Trestle environment, the lack of any confirmation or result dialog would most likely confuse the user, so rather than just disappearing, a command function without a return value (or with a null return value), will show a message on the screen that "Command 'Some Command' ran successfully".
If you really do not want the confirmation dialog (sometimes called a "fire and forget" command), you can return a special value that will inform Trestle to skip it. You can use the host.noResult() method to tell the Trestle interceptor to skip showing results. By the way, this method should not be used as a return value from a normal, non-command Dino function. It has no real purpose there. It won't harm anything but is not useful. You can find more about the special host object and the methods it makes available in More About the "host" Object.
def cmdTheMostSimpleCommand() {
return host.noResult();
}
The command shown above 1) does nothing, and 2) returns nothing. Now we are getting somewhere! Well not really. But as we'll see next, by collecting data from the user, we can get somewhere fast.